Linux 内核学习 ——— 系统调用
文章目录
系统调用
实验内容
在linux0.11上添加两个系统调用iam()
和whoami
,分别用于向内核空间存字符串和取字符串。
添加步骤
- 在
include/unistd.h
中添加系统调用号,并声明系统调用函数
#define __NR_iam 72
#define __NR_whoami 73
...
int iam(const char*name);
int whoami(char *name, unsigned int size);
- 在
kernel/system_call.s
中修改系统调用总个数,让系统感知到
nr_system_calls = 72 --> 74
- 在
include/sys.h
中添加到系统调用表
extern int sys_iam();
extern int sys_whoami();
...
fn_ptr sys_call_table[] = { sys_setup, ..., sys_iam, sys_whoami};
- 在
kernel
文件夹中新建文件who.c
实现系统调用
#include <string.h>
#include <errno.h>
#include <asm/segment.h>
#define MSGLEN 23
char msg[MSGLEN+1];
int sys_iam(const char * name){
int i=0;
char tmp[MSGLEN+1]={0};
for(;i<MSGLEN & ((tmp[i]=get_fs_byte(name+i))!='\0');i++);
printk("Get a string : %s and size is %d\n", tmp, i);
if(i>MSGLEN){
printk("String lenght over %d\n", MSGLEN);
return -(EINVAL);
}
strcpy(msg,tmp);
printk("Store %s to Kernel space\n");
return i;
}
int sys_whoami(char* name, unsigned int size){
int len = 0, i=0;
for(;msg[len]!='\0' & len<size;len++);
for(; i<size; put_fs_byte(msg[i], name+i), i++);
printk("Copy %s from kernel over!!!\n", msg);
return len;
}
测试系统调用
iam.c
#define __LIBRARY__
#include <unistd.h>
#include <stdio.h>
_syscall1(int, iam, const char*, name);
int main(int argc,char ** argv)
{
int len=0;
if(argc != 2){
printf("Usage: ./iam \"str\"\n");
return 0;
}
len = iam(argv[1]);
printf("store %d chars to kernel space\n", len);
return 0;
}
whoami.c
#define __LIBRARY__
#include <unistd.h>
#include <stdio.h>
_syscall2(int, whoami,char*,name,unsigned int,size);
int main(void){
char msg[1024]={0};
int len=0;
len=whoami(msg,1024);
printf("recive %d size str is %s",len, msg);
return 0;
}
实验结果
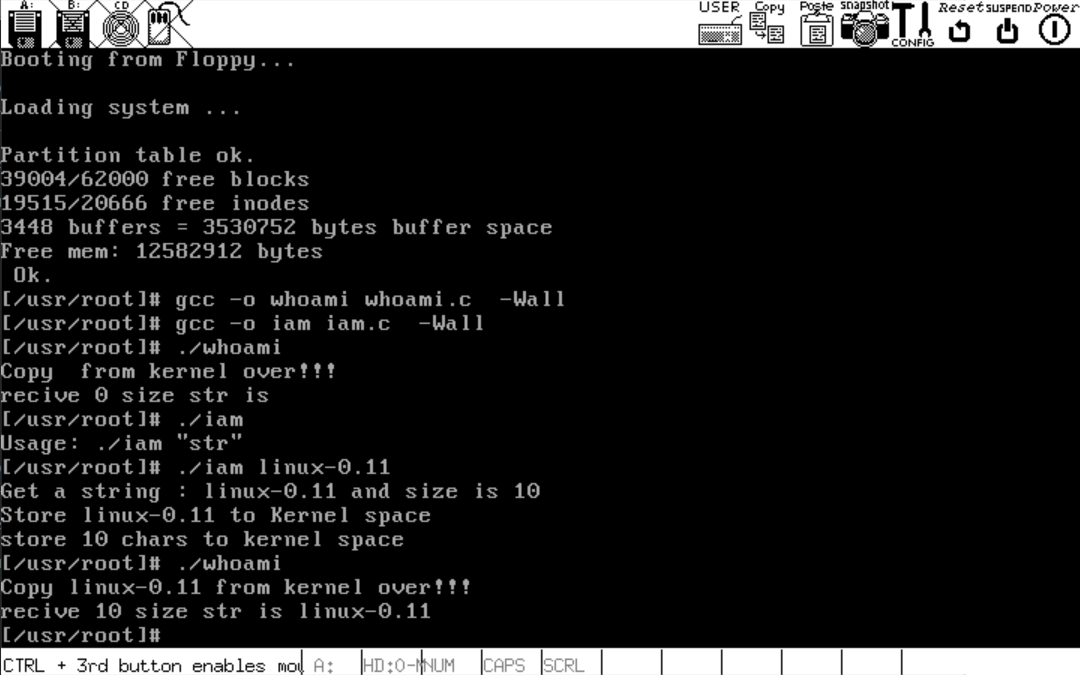
参考
[1] https://hoverwinter.gitbooks.io/hit-oslab-manual/sy2_syscall.html
[2] https://github.com/Wangzhike/HIT-Linux-0.11/blob/master/2-syscall/2-syscall.md